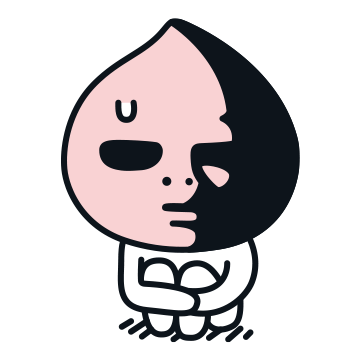
□생성자 : 객체가 생성될때 호출되는 생성자
(1) 디폴트생성자 : 객체가 생성될 때 가장 먼저 호출되는 생성자로, 만약 개발자가 명시하지 않아도 컴파일 시점에 자동 생성됨
예제 1) 클래스 생성
package pjt_test;
public class Newer_Class {
//Newer_Class 클래스의 생성자 지정
public Newer_Class() {
System.out.println("나는 생성자");
}
}
예제 1-1) 객체 생성
package pjt_test;
public class Newer_Class_Main {
public static void main(String[] args) {
//클래스에 대한 객체 생성과 동시에 생성자 바로 출력됨
Newer_Class newer_Class1 = new Newer_Class();
//*클래스에서 생성자를 지정안해도 객체 생성시 자동으로 클래스 생성자 생성됨
}
}
(2) 사용자 정의 생성자 : 객체가 생성될 때 가장 먼저 호출되는 생성자로, 개발자가 생성자를 별도로 지정한 것
예제 2) 클래스 생성
package pjt_test;
public class Newer_Class_Second {
//클래스에 대한 사용자 정의 생성자 생성
public Newer_Class_Second(String s, int[] iArr) {
System.out.println("나는 생성자. 스트링 매개변수와 배열을 받아요.");
//s는 매개변수로 String 값으로 객체 생성시 받은 String 값 출력
System.out.println("매개변수 s = " + s);
//iArr은 매개변수로 객체 생성시 받은 iArr 값이 저장된 주소 출력. 배열은 객체로 객체가 저장된 주소가 출력
System.out.println("매개변수 iArr(배열) = " + iArr);
}
}
예제 2-1) 객체 생성
package pjt_test;
public class Newer_Class_Second_Main {
public static void main(String[] args) {
int[] iArr = {10, 20, 30};
Newer_Class_Second Newer_Class2 = new Newer_Class_Second("Java", iArr);
}
}
□소멸자 : 객체가 소멸할때 호출되는 소멸자. 객체가 GC에 의하여 메모리에서 제거 될 때 finalize() 메서드가 호출됨
□ this : 클래스에서 지정한 전역변수를 특정하여 사용할 때 활용. '클래스 나 자신'이라고 생각하면 됨
tip1) 전역변수 : 클래스에서 사용할 변수. 객체가 생성되고 소멸될때까지 지속해서 메모리에 저장.
tip2) 지역변수 : 객체 생성시 호출부에서 생성자 생성과 동시에 받은 객체의 매개변수의 값을 지역변수로 저장.
예제 3) 클래스 생성
package pjt_test;
public class Newer_Class_Third {
//전역변수
//객체가 생성되고 소멸될때까지 지속해서 메모리에 저장되어있음
public int x;
public int y;
//생성자 생성
public Newer_Class_Third(){
System.out.println("나는 생성자");
}
//지역변수를 지닌 생성자 생성
//해당 생성자의 지역변수는 객체가 생성될 때 호출부에서 매개변수를 생성자의 지역변수로 토스함
public Newer_Class_Third(int x, int y) {
//this.전역변수(나자신) = 지역변수
//this는 해당 클래스를 의미
this.x = x;
this.y = y;
}
//클래스 메서드 생성
//매개변수로 받아 지역변수로 들어온 값
public void getInfo() {
System.out.println("x = " + this.x);
System.out.println("y = " + this.y);
}
}
예제 3-1) 객체 생성
package pjt_test;
public class Newer_Class_Third_Main {
public static void main(String[] args) {
//클래스를 활용하여 객체 생성
Newer_Class_Third Newer_Class3 = new Newer_Class_Third(10,20);
//생성된 객체에서 클래스의 메서드 호출
Newer_Class3.getInfo();
}
}
'Java > Java_basic' 카테고리의 다른 글
Step14. 데이터은닉과 Getter/Setter (0) | 2023.09.24 |
---|---|
Step13. 패키지와 Static (0) | 2023.09.24 |
Step11. 객체와 메모리 (0) | 2023.09.22 |
Step10. 객체 - 메서드와 접근자 (0) | 2023.09.22 |
Step9. 객체지향 프로그램 (0) | 2023.09.22 |